Note
Click here to download the full example code
Benchmark, comparison sklearn - forward-backward - classification¶
The benchmark compares the processing time between scikit-learn and onnxruntime-training on a logistic regression regression and a neural network for classification. It replicates the benchmark implemented in Benchmark, comparison scikit-learn - forward-backward.
First comparison: neural network¶
import warnings
import time
import numpy
import matplotlib.pyplot as plt
from pandas import DataFrame
from onnxruntime import get_device
from pyquickhelper.pycode.profiling import profile, profile2graph
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.neural_network import MLPClassifier
from mlprodict.onnx_conv import to_onnx
from mlprodict.plotting.text_plot import onnx_simple_text_plot
from mlprodict.onnx_tools.onnx_manipulations import select_model_inputs_outputs
from onnxcustom.utils.onnx_helper import onnx_rename_weights
from onnxcustom.training.optimizers_partial import (
OrtGradientForwardBackwardOptimizer)
from onnxcustom.training.sgd_learning_rate import LearningRateSGDNesterov
from onnxcustom.training.sgd_learning_loss import NegLogLearningLoss
from onnxcustom.training.sgd_learning_penalty import ElasticLearningPenalty
X, y = make_classification(1000, n_features=100, n_classes=2)
X = X.astype(numpy.float32)
y = y.astype(numpy.int64)
X_train, X_test, y_train, y_test = train_test_split(X, y)
Benchmark function.
def benchmark(X, y, skl_model, train_session, name, verbose=True):
"""
:param skl_model: model from scikit-learn
:param train_session: instance of OrtGradientForwardBackwardOptimizer
:param name: experiment name
:param verbose: to debug
"""
print("[benchmark] %s" % name)
begin = time.perf_counter()
skl_model.fit(X, y)
duration_skl = time.perf_counter() - begin
length_skl = len(skl_model.loss_curve_)
print("[benchmark] skl=%r iterations - %r seconds" % (
length_skl, duration_skl))
begin = time.perf_counter()
train_session.fit(X, y)
duration_ort = time.perf_counter() - begin
length_ort = len(train_session.train_losses_)
print("[benchmark] ort=%r iteration - %r seconds" % (
length_ort, duration_ort))
return dict(skl=duration_skl, ort=duration_ort, name=name,
iter_skl=length_skl, iter_ort=length_ort,
losses_skl=skl_model.loss_curve_,
losses_ort=train_session.train_losses_)
Common parameters and model
batch_size = 15
max_iter = 100
nn = MLPClassifier(hidden_layer_sizes=(50, 10), max_iter=max_iter,
solver='sgd', learning_rate_init=1e-1, alpha=1e-4,
n_iter_no_change=max_iter * 3, batch_size=batch_size,
nesterovs_momentum=True, momentum=0.9,
learning_rate="invscaling")
with warnings.catch_warnings():
warnings.simplefilter('ignore')
nn.fit(X_train, y_train)
Conversion to ONNX and trainer initialization It is slightly different from a regression model. Probabilities usually come from raw scores transformed through a function such as the sigmoid function. The gradient of the loss is computed against the raw scores because it is easier to compute than to let onnxruntime do it.
onx = to_onnx(nn, X_train[:1].astype(numpy.float32), target_opset=15,
options={'zipmap': False})
try:
print(onnx_simple_text_plot(onx))
except RuntimeError as e:
print("You should upgrade mlprodict.")
print(e)
Out:
opset: domain='' version=14
opset: domain='ai.onnx.ml' version=1
input: name='X' type=dtype('float32') shape=(0, 100)
init: name='coefficient' type=dtype('float32') shape=(5000,)
init: name='intercepts' type=dtype('float32') shape=(50,)
init: name='coefficient1' type=dtype('float32') shape=(500,)
init: name='intercepts1' type=dtype('float32') shape=(10,)
init: name='coefficient2' type=dtype('float32') shape=(10,)
init: name='intercepts2' type=dtype('float32') shape=(1,) -- array([0.28234306], dtype=float32)
init: name='unity' type=dtype('float32') shape=(1,) -- array([1.], dtype=float32)
init: name='classes' type=dtype('int32') shape=(2,) -- array([0, 1], dtype=int32)
init: name='shape_tensor' type=dtype('int64') shape=(1,) -- array([-1])
Cast(X, to=1) -> cast_input
MatMul(cast_input, coefficient) -> mul_result
Add(mul_result, intercepts) -> add_result
Relu(add_result) -> next_activations
MatMul(next_activations, coefficient1) -> mul_result1
Add(mul_result1, intercepts1) -> add_result1
Relu(add_result1) -> next_activations1
MatMul(next_activations1, coefficient2) -> mul_result2
Add(mul_result2, intercepts2) -> add_result2
Sigmoid(add_result2) -> out_activations_result
Sub(unity, out_activations_result) -> negative_class_proba
Concat(negative_class_proba, out_activations_result, axis=1) -> probabilities
ArgMax(probabilities, axis=1) -> argmax_output
ArrayFeatureExtractor(classes, argmax_output) -> array_feature_extractor_result
Reshape(array_feature_extractor_result, shape_tensor) -> reshaped_result
Cast(reshaped_result, to=7) -> label
output: name='label' type=dtype('int64') shape=(0,)
output: name='probabilities' type=dtype('float32') shape=(0, 2)
Raw scores are the input of operator Sigmoid.
onx = select_model_inputs_outputs(
onx, outputs=["add_result2"], infer_shapes=True)
print(onnx_simple_text_plot(onx))
Out:
opset: domain='' version=14
opset: domain='ai.onnx.ml' version=1
input: name='X' type=dtype('float32') shape=(0, 100)
init: name='coefficient' type=dtype('float32') shape=(5000,)
init: name='intercepts' type=dtype('float32') shape=(50,)
init: name='coefficient1' type=dtype('float32') shape=(500,)
init: name='intercepts1' type=dtype('float32') shape=(10,)
init: name='coefficient2' type=dtype('float32') shape=(10,)
init: name='intercepts2' type=dtype('float32') shape=(1,) -- array([0.28234306], dtype=float32)
Cast(X, to=1) -> cast_input
MatMul(cast_input, coefficient) -> mul_result
Add(mul_result, intercepts) -> add_result
Relu(add_result) -> next_activations
MatMul(next_activations, coefficient1) -> mul_result1
Add(mul_result1, intercepts1) -> add_result1
Relu(add_result1) -> next_activations1
MatMul(next_activations1, coefficient2) -> mul_result2
Add(mul_result2, intercepts2) -> add_result2
output: name='add_result2' type=dtype('float32') shape=(0, 1)
And the names are renamed to have them follow the
alphabetical order (see OrtGradientForwardBackward
).
onx = onnx_rename_weights(onx)
print(onnx_simple_text_plot(onx))
Out:
opset: domain='' version=14
opset: domain='ai.onnx.ml' version=1
input: name='X' type=dtype('float32') shape=(0, 100)
init: name='I0_coefficient' type=dtype('float32') shape=(5000,)
init: name='I1_intercepts' type=dtype('float32') shape=(50,)
init: name='I2_coefficient1' type=dtype('float32') shape=(500,)
init: name='I3_intercepts1' type=dtype('float32') shape=(10,)
init: name='I4_coefficient2' type=dtype('float32') shape=(10,)
init: name='I5_intercepts2' type=dtype('float32') shape=(1,) -- array([0.28234306], dtype=float32)
Cast(X, to=1) -> r7
MatMul(r7, I0_coefficient) -> r6
Add(r6, I1_intercepts) -> r5
Relu(r5) -> r4
MatMul(r4, I2_coefficient1) -> r3
Add(r3, I3_intercepts1) -> r2
Relu(r2) -> r1
MatMul(r1, I4_coefficient2) -> r0
Add(r0, I5_intercepts2) -> add_result2
output: name='add_result2' type=dtype('float32') shape=(0, 1)
We select the log loss (see NegLogLearningLoss
,
a simple regularization defined with ElasticLearningPenalty
,
and the Nesterov algorithm to update the weights with
LearningRateSGDNesterov
<onnxcustom.training.sgd_learning_rate.LearningRateSGDNesterov>.
train_session = OrtGradientForwardBackwardOptimizer(
onx, device='cpu', warm_start=False,
max_iter=max_iter, batch_size=batch_size,
learning_loss=NegLogLearningLoss(),
learning_rate=LearningRateSGDNesterov(
1e-7, nesterov=True, momentum=0.9),
learning_penalty=ElasticLearningPenalty(l1=0, l2=1e-4))
benches = [benchmark(X_train, y_train, nn, train_session, name='NN-CPU')]
Out:
[benchmark] NN-CPU
/var/lib/jenkins/workspace/onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sklearn/neural_network/_multilayer_perceptron.py:692: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (100) reached and the optimization hasn't converged yet.
warnings.warn(
[benchmark] skl=100 iterations - 12.829431453719735 seconds
[benchmark] ort=100 iteration - 16.35571615770459 seconds
Profiling¶
def clean_name(text):
pos = text.find('onnxruntime')
if pos >= 0:
return text[pos:]
pos = text.find('sklearn')
if pos >= 0:
return text[pos:]
pos = text.find('onnxcustom')
if pos >= 0:
return text[pos:]
pos = text.find('site-packages')
if pos >= 0:
return text[pos:]
return text
ps = profile(lambda: benchmark(X_train, y_train,
nn, train_session, name='NN-CPU'))[0]
root, nodes = profile2graph(ps, clean_text=clean_name)
text = root.to_text()
print(text)
Out:
[benchmark] NN-CPU
/var/lib/jenkins/workspace/onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sklearn/neural_network/_multilayer_perceptron.py:692: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (100) reached and the optimization hasn't converged yet.
warnings.warn(
[benchmark] skl=100 iterations - 14.402239209972322 seconds
[benchmark] ort=100 iteration - 19.739032081328332 seconds
filter -- 18 18 -- 0.00007 0.00018 -- /usr/local/lib/python3.9/logging/__init__.py:787:filter (filter)
filter -- 12 12 -- 0.00002 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:358:filter (filter)
filter -- 6 6 -- 0.00005 0.00006 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:491:filter (filter)
<built-in method builtins.isinstance> -- 18 18 -- 0.00001 0.00001 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.hasattr> -- 18 18 -- 0.00002 0.00002 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
acquire -- 30 30 -- 0.00007 0.00011 -- /usr/local/lib/python3.9/logging/__init__.py:892:acquire (acquire)
<method 'acquire' of '_thread.RLock' objects> -- 30 30 -- 0.00005 0.00005 -- ~:0:<method 'acquire' of '_thread.RLock' objects> (<method 'acquire' of '_thread.RLock' objects>)
release -- 30 30 -- 0.00006 0.00008 -- /usr/local/lib/python3.9/logging/__init__.py:899:release (release)
<method 'release' of '_thread.RLock' objects> -- 30 30 -- 0.00002 0.00002 -- ~:0:<method 'release' of '_thread.RLock' objects> (<method 'release' of '_thread.RLock' objects>)
emit -- 12 12 -- 0.00009 0.00113 -- /usr/local/lib/python3.9/logging/__init__.py:1067:emit (emit)
format -- 12 12 -- 0.00004 0.00059 -- /usr/local/lib/python3.9/logging/__init__.py:912:format (format)
format -- 12 12 -- 0.00010 0.00055 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:536:format (format)
format -- 12 12 -- 0.00008 0.00040 -- /usr/local/lib/python3.9/logging/__init__.py:646:format (format)
usesTime -- 12 12 -- 0.00002 0.00009 -- /usr/local/lib/python3.9/logging/__init__.py:624:usesTime (usesTime)
usesTime -- 12 12 -- 0.00004 0.00006 -- /usr/local/lib/python3.9/logging/__init__.py:417:usesTime (usesTime)
<method 'find' of 'str' objects> -- 12 12 -- 0.00002 0.00002 -- ~:0:<method 'find' of 'str' objects> (<method 'find' of 'str' objects>)
formatMessage -- 12 12 -- 0.00002 0.00009 -- /usr/local/lib/python3.9/logging/__init__.py:630:formatMessage (formatMessage)
format -- 12 12 -- 0.00002 0.00007 -- /usr/local/lib/python3.9/logging/__init__.py:428:format (format)
_format -- 12 12 -- 0.00005 0.00005 -- /usr/local/lib/python3.9/logging/__init__.py:425:_format (_format)
getMessage -- 12 12 -- 0.00007 0.00014 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:96:getMessage (getMessage)
getMessage -- 12 12 -- 0.00006 0.00006 -- /usr/local/lib/python3.9/logging/__init__.py:354:getMessage (getMessage)
<built-in method builtins.getattr> -- 12 12 -- 0.00001 0.00001 -- ~:0:<built-in method builtins.getattr> (<built-in method builtins.getattr>) +++
colorize -- 2 2 -- 0.00002 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/console.py:85:colorize (colorize)
escseq -- 4 4 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/console.py:86:escseq (escseq)
<built-in method builtins.getattr> -- 12 12 -- 0.00001 0.00001 -- ~:0:<built-in method builtins.getattr> (<built-in method builtins.getattr>) +++
flush -- 12 12 -- 0.00007 0.00039 -- /usr/local/lib/python3.9/logging/__init__.py:1056:flush (flush)
acquire -- 12 12 -- 0.00002 0.00004 -- /usr/local/lib/python3.9/logging/__init__.py:892:acquire (acquire) +++
release -- 12 12 -- 0.00003 0.00004 -- /usr/local/lib/python3.9/logging/__init__.py:899:release (release) +++
flush -- 6 6 -- 0.00002 0.00023 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:562:flush (flush)
<method 'flush' of '_io.TextIOWrapper' objects> -- 6 6 -- 0.00019 0.00019 -- ~:0:<method 'flush' of '_io.TextIOWrapper' objects> (<method 'flush' of '_io.TextIOWrapper' objects>)
<built-in method builtins.hasattr> -- 12 12 -- 0.00001 0.00001 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
write -- 6 6 -- 0.00003 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:554:write (write)
write -- 6 6 -- 0.00003 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:572:write (write)
isEnabledFor -- 12 12 -- 0.00002 0.00002 -- /usr/local/lib/python3.9/logging/__init__.py:1677:isEnabledFor (isEnabledFor)
inner -- 6 6 -- 0.00002 0.00002 -- /usr/local/lib/python3.9/typing.py:256:inner (inner)
cast -- 6 6 -- 0.00000 0.00000 -- /usr/local/lib/python3.9/typing.py:1326:cast (cast)
simplefilter -- 11 11 -- 0.00005 0.00026 -- /usr/local/lib/python3.9/warnings.py:165:simplefilter (simplefilter)
_add_filter -- 11 11 -- 0.00011 0.00020 -- /usr/local/lib/python3.9/warnings.py:181:_add_filter (_add_filter)
<method 'insert' of 'list' objects> -- 11 11 -- 0.00002 0.00002 -- ~:0:<method 'insert' of 'list' objects> (<method 'insert' of 'list' objects>)
<method 'remove' of 'list' objects> -- 11 11 -- 0.00007 0.00007 -- ~:0:<method 'remove' of 'list' objects> (<method 'remove' of 'list' objects>)
<built-in method _warnings._filters_mutated> -- 11 11 -- 0.00001 0.00001 -- ~:0:<built-in method _warnings._filters_mutated> (<built-in method _warnings._filters_mutated>) +++
<built-in method builtins.isinstance> -- 11 11 -- 0.00001 0.00001 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
__init__ -- 2 2 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/warnings.py:403:__init__ (__init__)
__init__ -- 11 11 -- 0.00004 0.00004 -- /usr/local/lib/python3.9/warnings.py:437:__init__ (__init__)
__enter__ -- 11 11 -- 0.00009 0.00010 -- /usr/local/lib/python3.9/warnings.py:458:__enter__ (__enter__)
<built-in method _warnings._filters_mutated> -- 11 11 -- 0.00001 0.00001 -- ~:0:<built-in method _warnings._filters_mutated> (<built-in method _warnings._filters_mutated>) +++
__exit__ -- 11 11 -- 0.00006 0.00006 -- /usr/local/lib/python3.9/warnings.py:477:__exit__ (__exit__)
<built-in method _warnings._filters_mutated> -- 11 11 -- 0.00001 0.00001 -- ~:0:<built-in method _warnings._filters_mutated> (<built-in method _warnings._filters_mutated>) +++
any -- 2 2 -- 0.00002 0.00018 -- <__array_function__ internals>:177:any (any)
_any_dispatcher -- 2 2 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:2300:_any_dispatcher (_any_dispatcher)
<built-in method numpy.core....implement_array_function> -- 2 2 -- 0.00003 0.00015 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
clip -- 5001 5001 -- 0.03248 0.99188 -- <__array_function__ internals>:177:clip (clip)
_clip_dispatcher -- 5001 5001 -- 0.00547 0.00547 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:2079:_clip_dispatcher (_clip_dispatcher)
<built-in method numpy.core....implement_array_function> -- 5001 5001 -- 0.07215 0.95393 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
unique -- 106 106 -- 0.00064 0.01184 -- <__array_function__ internals>:177:unique (unique)
_unique_dispatcher -- 106 106 -- 0.00011 0.00011 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/lib/arraysetops.py:133:_unique_dispatcher (_unique_dispatcher)
<built-in method numpy.core....implement_array_function> -- 106 106 -- 0.00079 0.01109 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
empty_like -- 7 7 -- 0.00004 0.00011 -- <__array_function__ internals>:177:empty_like (empty_like)
empty_like -- 7 7 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/multiarray.py:80:empty_like (empty_like)
<lambda> -- 1 1 -- 0.00001 34.14365 -- onnxcustom/onnxcustom_UT_39_std/_doc/examples/plot_orttraining_benchmark_fwbw_cls.py:168:<lambda> (<lambda>)
benchmark -- 1 1 -- 0.00013 34.14364 -- onnxcustom/onnxcustom_UT_39_std/_doc/examples/plot_orttraining_benchmark_fwbw_cls.py:50:benchmark (benchmark)
fit -- 1 1 -- 0.00553 19.73899 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:263:fit (fit)
__init__ -- 1 1 -- 0.00005 0.00009 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/data_loader.py:31:__init__ (__init__)
get_ort_device -- 1 1 -- 0.00000 0.00000 -- onnxruntime_helper.py:63:get_ort_device (get_ort_device)
numpy_to_ort_value -- 2 2 -- 0.00001 0.00003 -- onnxruntime_helper.py:134:numpy_to_ort_value (numpy_to_ort_value) +++
needs_grad -- 3 3 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:99:needs_grad (needs_grad)
needs_grad -- 3 3 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:337:needs_grad (needs_grad)
get_full_state -- 101 101 -- 0.00073 0.00327 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:147:get_full_state (get_full_state) +++
set_state -- 4 4 -- 0.00033 0.00112 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:196:set_state (set_state)
_get_att_state -- 4 4 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:139:_get_att_state (_get_att_state) +++
numpy_to_ort_value -- 24 24 -- 0.00008 0.00041 -- onnxruntime_helper.py:134:numpy_to_ort_value (numpy_to_ort_value) +++
<built-in method numpy.zeros> -- 12 12 -- 0.00007 0.00007 -- ~:0:<built-in method numpy.zeros> (<built-in method numpy.zeros>) +++
<method 'append' of 'list' objects> -- 56 56 -- 0.00003 0.00003 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>) +++
<built-in method builtins.hasattr> -- 4 4 -- 0.00019 0.00019 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<built-in method builtins.isinstance> -- 24 24 -- 0.00002 0.00002 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<listcomp> -- 1 1 -- 0.00006 0.00360 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:311:<listcomp> (<listcomp>)
get_initializer -- 7 7 -- 0.00020 0.00354 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:280:get_initializer (get_initializer) +++
<listcomp> -- 1 1 -- 0.00005 0.00285 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:315:<listcomp> (<listcomp>)
get_initializer -- 7 7 -- 0.00020 0.00280 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:280:get_initializer (get_initializer) +++
_iteration -- 100 100 -- 1.79248 19.68066 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:397:_iteration (_iteration)
iter_ortvalue -- 5100 5100 -- 0.12190 0.66953 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/data_loader.py:147:iter_ortvalue (iter_ortvalue)
_next_iter -- 5000 5000 -- 0.03942 0.35568 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/data_loader.py:98:_next_iter (_next_iter)
<method 'randint'...mState' objects> -- 5000 5000 -- 0.30333 0.30333 -- ~:0:<method 'randint' of 'numpy.random.mtrand.RandomState' objects> (<method 'randint' of 'numpy.random.mtrand.RandomState' objects>)
<built-in method builtins.len> -- 5000 5000 -- 0.00767 0.01293 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
numpy_to_ort_value -- 10000 10000 -- 0.03210 0.15669 -- onnxruntime_helper.py:134:numpy_to_ort_value (numpy_to_ort_value) +++
<built-in method builtins.len> -- 5200 5200 -- 0.01746 0.03525 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
forward -- 5000 5000 -- 1.14285 1.50598 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:655:forward (forward)
input_to_ort -- 5000 5000 -- 0.21496 0.30590 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:578:input_to_ort (input_to_ort) +++
save_for_backward -- 5000 5000 -- 0.04654 0.04654 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:630:save_for_backward (save_for_backward)
<method 'append' of 'list' objects> -- 5000 5000 -- 0.01069 0.01069 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>) +++
backward -- 5000 5000 -- 1.56197 1.76075 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:737:backward (backward)
input_to_ort -- 5000 5000 -- 0.13888 0.18149 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:578:input_to_ort (input_to_ort) +++
saved_tensors -- 5000 5000 -- 0.00685 0.00685 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:642:saved_tensors (saved_tensors)
<method 'pop' of 'list' objects> -- 5000 5000 -- 0.01044 0.01044 -- ~:0:<method 'pop' of 'list' objects> (<method 'pop' of 'list' objects>)
loss_gradient -- 5000 5000 -- 0.25857 1.34957 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_loss.py:61:loss_gradient (loss_gradient)
clear_binding_inputs -- 5000 5000 -- 0.02139 0.05011 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:147:clear_binding_inputs (clear_binding_inputs)
_cache_in_clear -- 5000 5000 -- 0.02161 0.02872 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:136:_cache_in_clear (_cache_in_clear)
<built-in method builtins.id> -- 5000 5000 -- 0.00711 0.00711 -- ~:0:<built-in method builtins.id> (<built-in method builtins.id>) +++
_bind_input_ortvalue -- 10000 10000 -- 0.07677 0.33085 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:179:_bind_input_ortvalue (_bind_input_ortvalue) +++
_call_iobinding -- 5000 5000 -- 0.69954 0.69954 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_loss.py:58:_call_iobinding (_call_iobinding)
<built-in method builtins.hasattr> -- 10000 10000 -- 0.01049 0.01049 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
penalty_loss -- 5000 5000 -- 0.29701 2.03358 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_penalty.py:168:penalty_loss (penalty_loss)
_bind_input_ortvalue -- 35000 35000 -- 0.17349 0.56303 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:179:_bind_input_ortvalue (_bind_input_ortvalue) +++
_bind_output_ortvalue -- 5000 5000 -- 0.03842 0.14657 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:225:_bind_output_ortvalue (_bind_output_ortvalue) +++
_call_iobinding -- 5000 5000 -- 1.01379 1.01379 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_penalty.py:27:_call_iobinding (_call_iobinding) +++
<built-in method builtins.hasattr> -- 10000 10000 -- 0.00876 0.00876 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<built-in method builtins.len> -- 10000 10000 -- 0.00442 0.00442 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
update_weights -- 30000 30000 -- 0.69175 3.17189 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_penalty.py:198:update_weights (update_weights)
_bind_input_ortvalue -- 30000 30000 -- 0.15196 0.47831 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:179:_bind_input_ortvalue (_bind_input_ortvalue) +++
_bind_output_ortvalue -- 30000 30000 -- 0.13885 0.42685 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:225:_bind_output_ortvalue (_bind_output_ortvalue) +++
_call_iobinding -- 30000 30000 -- 1.53237 1.53237 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_penalty.py:27:_call_iobinding (_call_iobinding) +++
<built-in method builtins.hasattr> -- 60000 60000 -- 0.04260 0.04260 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
update_weights -- 30000 30000 -- 1.77980 7.32196 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:392:update_weights (update_weights)
_bind_input_ortvalue -- 150000 150000 -- 0.70486 2.12471 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:179:_bind_input_ortvalue (_bind_input_ortvalue) +++
_bind_output_ortvalue -- 60000 60000 -- 0.32183 0.86631 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:225:_bind_output_ortvalue (_bind_output_ortvalue) +++
_call_iobinding -- 30000 30000 -- 1.73590 1.73590 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:33:_call_iobinding (_call_iobinding)
value -- 30000 30000 -- 0.03628 0.03628 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:186:value (value) +++
<built-in method on...tvalue_from_numpy> -- 60000 60000 -- 0.72955 0.72955 -- ~:0:<built-in method onnxruntime.capi.onnxruntime_pybind11_state.ortvalue_from_numpy> (<built-in method onnxruntime.capi.onnxruntime_pybind11_state.ortvalue_from_numpy>) +++
<built-in method builtins.hasattr> -- 60000 60000 -- 0.04942 0.04942 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<method 'mean' of 'numpy.ndarray' objects> -- 100 100 -- 0.00068 0.01596 -- ~:0:<method 'mean' of 'numpy.ndarray' objects> (<method 'mean' of 'numpy.ndarray' objects>)
_mean -- 100 100 -- 0.00718 0.01528 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:162:_mean (_mean) +++
<built-in method numpy.array> -- 100 100 -- 0.00854 0.00854 -- ~:0:<built-in method numpy.array> (<built-in method numpy.array>) +++
<method 'append' of 'list' objects> -- 5000 5000 -- 0.00603 0.00603 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>) +++
<built-in method builtins.len> -- 30100 30100 -- 0.04438 0.04438 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
_create_training_session -- 1 1 -- 0.00003 0.03696 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:626:_create_training_session (_create_training_session)
__init__ -- 1 1 -- 0.00017 0.03689 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:59:__init__ (__init__)
<listcomp> -- 1 1 -- 0.00003 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:96:<listcomp> (<listcomp>)
<listcomp> -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:99:<listcomp> (<listcomp>)
<listcomp> -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:118:<listcomp> (<listcomp>)
_init_next -- 1 1 -- 0.00021 0.03665 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:171:_init_next (_init_next)
<listcomp> -- 1 1 -- 0.00003 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:181:<listcomp> (<listcomp>)
<listcomp> -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:183:<listcomp> (<listcomp>)
<listcomp> -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:186:<listcomp> (<listcomp>)
_create_onnx_graphs -- 1 1 -- 0.00768 0.03640 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:301:_create_onnx_graphs (_create_onnx_graphs)
<listcomp> -- 1 1 -- 0.00002 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:413:<listcomp> (<listcomp>)
<listcomp> -- 1 1 -- 0.00002 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:414:<listcomp> (<listcomp>)
<listcomp> -- 1 1 -- 0.00003 0.00004 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:416:<listcomp> (<listcomp>)
_provider_nam..._device_type -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:271:_provider_name_to_device_type (_provider_name_to_device_type) +++
<listcomp> -- 1 1 -- 0.00008 0.00011 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:421:<listcomp> (<listcomp>)
_provider_nam..._device_type -- 7 7 -- 0.00001 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:271:_provider_name_to_device_type (_provider_name_to_device_type) +++
<listcomp> -- 1 1 -- 0.00001 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:427:<listcomp> (<listcomp>)
_provider_nam..._device_type -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:271:_provider_name_to_device_type (_provider_name_to_device_type) +++
<listcomp> -- 1 1 -- 0.00003 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:496:<listcomp> (<listcomp>)
<listcomp> -- 1 1 -- 0.00002 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:497:<listcomp> (<listcomp>)
load_model -- 2 2 -- 0.00002 0.00118 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/onnx/__init__.py:107:load_model (load_model)
_load_bytes -- 2 2 -- 0.00005 0.00007 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/onnx/__init__.py:30:_load_bytes (_load_bytes)
inner -- 4 4 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/typing.py:256:inner (inner) +++
cast -- 4 4 -- 0.00000 0.00000 -- /usr/local/lib/python3.9/typing.py:1326:cast (cast) +++
_get_file_path -- 2 2 -- 0.00000 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/onnx/__init__.py:50:_get_file_path (_get_file_path)
load_model_from_string -- 2 2 -- 0.00003 0.00109 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/onnx/__init__.py:147:load_model_from_string (load_model_from_string)
_deserialize -- 2 2 -- 0.00004 0.00106 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/onnx/__init__.py:81:_deserialize (_deserialize)
inner -- 2 2 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/typing.py:256:inner (inner) +++
cast -- 2 2 -- 0.00000 0.00000 -- /usr/local/lib/python3.9/typing.py:1326:cast (cast) +++
<method '...objects> -- 2 2 -- 0.00100 0.00100 -- ~:0:<method 'ParseFromString' of 'google.protobuf.pyext._message.CMessage' objects> (<method 'ParseFromString' of 'google.protobuf.pyext._message.CMessage' objects>)
get_inputs -- 1 1 -- 0.00000 0.00000 -- onnxruntime/capi/onnxruntime_inference_collection.py:111:get_inputs (get_inputs)
get_outputs -- 1 1 -- 0.00000 0.00000 -- onnxruntime/capi/onnxruntime_inference_collection.py:115:get_outputs (get_outputs)
__init__ -- 2 2 -- 0.00009 0.02701 -- onnxruntime/capi/onnxruntime_inference_collection.py:283:__init__ (__init__)
get -- 2 2 -- 0.00002 0.00008 -- /usr/local/lib/python3.9/_collections_abc.py:675:get (get)
__getitem__ -- 2 2 -- 0.00003 0.00006 -- /usr/local/lib/python3.9/os.py:674:__getitem__ (__getitem__)
encode -- 2 2 -- 0.00002 0.00003 -- /usr/local/lib/python3.9/os.py:754:encode (encode)
__init__ -- 2 2 -- 0.00001 0.00001 -- onnxruntime/capi/onnxruntime_inference_collection.py:101:__init__ (__init__)
_create_inference_session -- 2 2 -- 0.02661 0.02682 -- onnxruntime/capi/onnxruntime_inference_collection.py:346:_create_inference_session (_create_inference_session)
check_and_n...vider_args -- 2 2 -- 0.00011 0.00019 -- onnxruntime/capi/onnxruntime_inference_collection.py:25:check_and_normalize_provider_args (check_and_normalize_provider_args)
set_provider_options -- 2 2 -- 0.00002 0.00002 -- onnxruntime/capi/onnxruntime_inference_collection.py:53:set_provider_options (set_provider_options)
<dictcomp> -- 2 2 -- 0.00000 0.00000 -- onnxruntime/capi/onnxruntime_inference_collection.py:62:<dictcomp> (<dictcomp>)
<listcomp> -- 2 2 -- 0.00001 0.00001 -- onnxruntime/capi/onnxruntime_inference_collection.py:75:<listcomp> (<listcomp>)
<listcomp> -- 2 2 -- 0.00001 0.00001 -- onnxruntime/capi/onnxruntime_inference_collection.py:78:<listcomp> (<listcomp>)
<method 'Serial...sage' objects> -- 1 1 -- 0.00024 0.00024 -- ~:0:<method 'SerializeToString' of 'google.protobuf.pyext._message.CMessage' objects> (<method 'SerializeToString' of 'google.protobuf.pyext._message.CMessage' objects>)
<built-in method builtins.len> -- 16 16 -- 0.00001 0.00001 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
new_instance -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:219:new_instance (new_instance)
__init__ -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:521:__init__ (__init__)
device_to_providers -- 1 1 -- 0.00003 0.00003 -- onnxruntime_helper.py:150:device_to_providers (device_to_providers)
value -- 100 100 -- 0.00013 0.00013 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:186:value (value) +++
init_learning_rate -- 1 1 -- 0.00000 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:348:init_learning_rate (init_learning_rate)
init_learning_rate -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:205:init_learning_rate (init_learning_rate)
update_learning_rate -- 100 100 -- 0.00043 0.00375 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:358:update_learning_rate (update_learning_rate)
update_learning_rate -- 100 100 -- 0.00332 0.00332 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:226:update_learning_rate (update_learning_rate)
proto_type_to_dtype -- 6 6 -- 0.00002 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/utils/onnx_helper.py:61:proto_type_to_dtype (proto_type_to_dtype)
<method 'randn' of 'num....RandomState' objects> -- 6 6 -- 0.00075 0.00075 -- ~:0:<method 'randn' of 'numpy.random.mtrand.RandomState' objects> (<method 'randn' of 'numpy.random.mtrand.RandomState' objects>)
<method 'append' of 'list' objects> -- 107 107 -- 0.00010 0.00010 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>) +++
<built-in method builtins.len> -- 108 108 -- 0.00007 0.00007 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
fit -- 1 1 -- 0.00002 14.40223 -- sklearn/neural_network/_multilayer_perceptron.py:735:fit (fit)
_fit -- 1 1 -- 0.00014 14.40221 -- sklearn/neural_network/_multilayer_perceptron.py:376:_fit (_fit)
any -- 1 1 -- 0.00001 0.00010 -- <__array_function__ internals>:177:any (any) +++
_initialize -- 1 1 -- 0.00006 0.00065 -- sklearn/neural_network/_multilayer_perceptron.py:319:_initialize (_initialize)
is_classifier -- 1 1 -- 0.00000 0.00000 -- sklearn/base.py:960:is_classifier (is_classifier)
_init_coef -- 3 3 -- 0.00010 0.00058 -- sklearn/neural_network/_multilayer_perceptron.py:359:_init_coef (_init_coef)
<method 'uniform'...mState' objects> -- 6 6 -- 0.00041 0.00041 -- ~:0:<method 'uniform' of 'numpy.random.mtrand.RandomState' objects> (<method 'uniform' of 'numpy.random.mtrand.RandomState' objects>)
<listcomp> -- 1 1 -- 0.00001 0.00003 -- sklearn/neural_network/_multilayer_perceptron.py:416:<listcomp> (<listcomp>)
<listcomp> -- 1 1 -- 0.00001 0.00003 -- sklearn/neural_network/_multilayer_perceptron.py:421:<listcomp> (<listcomp>)
_validate_hyperparameters -- 1 1 -- 0.00002 0.00002 -- sklearn/neural_network/_multilayer_perceptron.py:445:_validate_hyperparameters (_validate_hyperparameters)
_fit_stochastic -- 1 1 -- 0.29894 14.39679 -- sklearn/neural_network/_multilayer_perceptron.py:553:_fit_stochastic (_fit_stochastic)
clip -- 1 1 -- 0.00001 0.00022 -- <__array_function__ internals>:177:clip (clip) +++
_backprop -- 5000 5000 -- 0.69186 9.48382 -- sklearn/neural_network/_multilayer_perceptron.py:240:_backprop (_backprop)
dot -- 15000 15000 -- 0.06738 0.29225 -- <__array_function__ internals>:177:dot (dot)
dot -- 15000 15000 -- 0.01250 0.01250 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/multiarray.py:736:dot (dot)
<built-in metho...rray_function> -- 15000 15000 -- 0.21237 0.21237 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
inplace_relu_derivative -- 10000 10000 -- 0.43888 0.43888 -- sklearn/neural_network/_base.py:132:inplace_relu_derivative (inplace_relu_derivative)
binary_log_loss -- 5000 5000 -- 0.90724 2.26017 -- sklearn/neural_network/_base.py:205:binary_log_loss (binary_log_loss)
clip -- 5000 5000 -- 0.03247 0.99166 -- <__array_function__ internals>:177:clip (clip) +++
__new__ -- 5000 5000 -- 0.04389 0.05317 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/getlimits.py:457:__new__ (__new__)
<method 'get'...ct' objects> -- 5000 5000 -- 0.00928 0.00928 -- ~:0:<method 'get' of 'dict' objects> (<method 'get' of 'dict' objects>) +++
<method 'sum' o...rray' objects> -- 10000 10000 -- 0.04512 0.30810 -- ~:0:<method 'sum' of 'numpy.ndarray' objects> (<method 'sum' of 'numpy.ndarray' objects>)
_sum -- 10000 10000 -- 0.02075 0.26298 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:46:_sum (_sum)
<method 're...' objects> -- 10000 10000 -- 0.24222 0.24222 -- ~:0:<method 'reduce' of 'numpy.ufunc' objects> (<method 'reduce' of 'numpy.ufunc' objects>) +++
_forward_pass -- 5000 5000 -- 0.50466 1.61987 -- sklearn/neural_network/_multilayer_perceptron.py:118:_forward_pass (_forward_pass)
inplace_logistic -- 5000 5000 -- 0.08381 0.08381 -- sklearn/neural_network/_base.py:25:inplace_logistic (inplace_logistic)
inplace_relu -- 10000 10000 -- 0.34447 0.34447 -- sklearn/neural_network/_base.py:47:inplace_relu (inplace_relu)
safe_sparse_dot -- 15000 15000 -- 0.64332 0.68693 -- sklearn/utils/extmath.py:120:safe_sparse_dot (safe_sparse_dot) +++
_compute_loss_grad -- 15000 15000 -- 1.33320 3.81843 -- sklearn/neural_network/_multilayer_perceptron.py:176:_compute_loss_grad (_compute_loss_grad)
mean -- 15000 15000 -- 0.07532 1.75372 -- <__array_function__ internals>:177:mean (mean)
_mean_dispatcher -- 15000 15000 -- 0.01432 0.01432 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:3351:_mean_dispatcher (_mean_dispatcher)
<built-in met...ay_function> -- 15000 15000 -- 0.06828 1.66409 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
safe_sparse_dot -- 15000 15000 -- 0.68659 0.73150 -- sklearn/utils/extmath.py:120:safe_sparse_dot (safe_sparse_dot) +++
safe_sparse_dot -- 10000 10000 -- 0.29789 0.32663 -- sklearn/utils/extmath.py:120:safe_sparse_dot (safe_sparse_dot) +++
<method 'ravel' o...darray' objects> -- 15000 15000 -- 0.03573 0.03573 -- ~:0:<method 'ravel' of 'numpy.ndarray' objects> (<method 'ravel' of 'numpy.ndarray' objects>) +++
_update_no_improvement_count -- 100 100 -- 0.00079 0.00079 -- sklearn/neural_network/_multilayer_perceptron.py:706:_update_no_improvement_count (_update_no_improvement_count)
update_params -- 5000 5000 -- 0.45580 3.48865 -- sklearn/neural_network/_stochastic_optimizers.py:29:update_params (update_params)
<genexpr> -- 35000 35000 -- 0.03327 0.03327 -- sklearn/neural_network/_stochastic_optimizers.py:43:<genexpr> (<genexpr>)
_get_updates -- 5000 5000 -- 0.10949 2.99958 -- sklearn/neural_network/_stochastic_optimizers.py:169:_get_updates (_get_updates)
<listcomp> -- 5000 5000 -- 1.47044 1.47044 -- sklearn/neural_network/_stochastic_optimizers.py:183:<listcomp> (<listcomp>)
<listcomp> -- 5000 5000 -- 1.41965 1.41965 -- sklearn/neural_network/_stochastic_optimizers.py:190:<listcomp> (<listcomp>)
__init__ -- 1 1 -- 0.00002 0.00042 -- sklearn/neural_network/_stochastic_optimizers.py:121:__init__ (__init__)
__init__ -- 1 1 -- 0.00001 0.00001 -- sklearn/neural_network/_stochastic_optimizers.py:25:__init__ (__init__)
<listcomp> -- 1 1 -- 0.00003 0.00040 -- sklearn/neural_network/_stochastic_optimizers.py:136:<listcomp> (<listcomp>)
zeros_like -- 6 6 -- 0.00002 0.00037 -- <__array_function__ internals>:177:zeros_like (zeros_like)
_zeros_like_dispatcher -- 6 6 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/numeric.py:72:_zeros_like_dispatcher (_zeros_like_dispatcher)
<built-in met...ay_function> -- 6 6 -- 0.00003 0.00034 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
iteration_ends -- 100 100 -- 0.00124 0.00124 -- sklearn/neural_network/_stochastic_optimizers.py:138:iteration_ends (iteration_ends)
_safe_indexing -- 5000 5000 -- 0.05562 1.01595 -- sklearn/utils/__init__.py:307:_safe_indexing (_safe_indexing) +++
shuffle -- 100 100 -- 0.00119 0.05295 -- sklearn/utils/__init__.py:602:shuffle (shuffle)
resample -- 100 100 -- 0.00322 0.05176 -- sklearn/utils/__init__.py:452:resample (resample)
<listcomp> -- 100 100 -- 0.00040 0.00072 -- sklearn/utils/__init__.py:593:<listcomp> (<listcomp>)
isspmatrix -- 100 100 -- 0.00016 0.00031 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix) +++
<listcomp> -- 100 100 -- 0.00041 0.01952 -- sklearn/utils/__init__.py:594:<listcomp> (<listcomp>)
_safe_indexing -- 100 100 -- 0.00101 0.01911 -- sklearn/utils/__init__.py:307:_safe_indexing (_safe_indexing) +++
check_consistent_length -- 100 100 -- 0.00121 0.01719 -- sklearn/utils/validation.py:318:check_consistent_length (check_consistent_length) +++
check_random_state -- 100 100 -- 0.00096 0.00246 -- sklearn/utils/validation.py:1043:check_random_state (check_random_state) +++
<method 'shuffl...tate' objects> -- 100 100 -- 0.00679 0.00679 -- ~:0:<method 'shuffle' of 'numpy.random.mtrand.RandomState' objects> (<method 'shuffle' of 'numpy.random.mtrand.RandomState' objects>)
<built-in method numpy.arange> -- 100 100 -- 0.00147 0.00147 -- ~:0:<built-in method numpy.arange> (<built-in method numpy.arange>) +++
<built-in metho...ltins.hasattr> -- 100 100 -- 0.00023 0.00023 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<built-in method builtins.len> -- 200 200 -- 0.00017 0.00017 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
gen_batches -- 5100 5100 -- 0.05145 0.05250 -- sklearn/utils/__init__.py:712:gen_batches (gen_batches)
<built-in method ...tins.isinstance> -- 100 100 -- 0.00034 0.00105 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<method 'append' of 'list' objects> -- 100 100 -- 0.00013 0.00013 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>) +++
<built-in method _warnings.warn> -- 1 1 -- 0.00007 0.00117 -- ~:0:<built-in method _warnings.warn> (<built-in method _warnings.warn>)
_showwarnmsg -- 1 1 -- 0.00002 0.00109 -- /usr/local/lib/python3.9/warnings.py:96:_showwarnmsg (_showwarnmsg)
_showwarning -- 1 1 -- 0.00001 0.00108 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx_gallery/gen_rst.py:473:_showwarning (_showwarning)
formatwarning -- 1 1 -- 0.00001 0.00006 -- /usr/local/lib/python3.9/warnings.py:15:formatwarning (formatwarning)
_formatwarnmsg_impl -- 1 1 -- 0.00004 0.00005 -- /usr/local/lib/python3.9/warnings.py:35:_formatwarnmsg_impl (_formatwarnmsg_impl)
getline -- 1 1 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/linecache.py:26:getline (getline)
getlines -- 1 1 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/linecache.py:36:getlines (getlines)
__init__ -- 1 1 -- 0.00000 0.00000 -- /usr/local/lib/python3.9/warnings.py:403:__init__ (__init__) +++
write -- 1 1 -- 0.00002 0.00100 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx_gallery/gen_rst.py:81:write (write) +++
__init__ -- 1 1 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/warnings.py:403:__init__ (__init__) +++
_validate_input -- 1 1 -- 0.00007 0.00442 -- sklearn/neural_network/_multilayer_perceptron.py:1099:_validate_input (_validate_input)
_validate_data -- 1 1 -- 0.00003 0.00117 -- sklearn/base.py:495:_validate_data (_validate_data)
_check_n_features -- 1 1 -- 0.00001 0.00002 -- sklearn/base.py:359:_check_n_features (_check_n_features)
_num_features -- 1 1 -- 0.00001 0.00002 -- sklearn/utils/validation.py:201:_num_features (_num_features)
_check_feature_names -- 1 1 -- 0.00000 0.00001 -- sklearn/base.py:405:_check_feature_names (_check_feature_names)
_get_feature_names -- 1 1 -- 0.00000 0.00000 -- sklearn/utils/validation.py:1653:_get_feature_names (_get_feature_names)
check_X_y -- 1 1 -- 0.00001 0.00111 -- sklearn/utils/validation.py:845:check_X_y (check_X_y)
check_consistent_length -- 1 1 -- 0.00001 0.00024 -- sklearn/utils/validation.py:318:check_consistent_length (check_consistent_length) +++
check_array -- 1 1 -- 0.00006 0.00066 -- sklearn/utils/validation.py:494:check_array (check_array) +++
_check_y -- 1 1 -- 0.00001 0.00020 -- sklearn/utils/validation.py:986:_check_y (_check_y)
check_array -- 1 1 -- 0.00004 0.00019 -- sklearn/utils/validation.py:494:check_array (check_array) +++
__init__ -- 1 1 -- 0.00000 0.00000 -- sklearn/preprocessing/_label.py:258:__init__ (__init__)
fit -- 1 1 -- 0.00002 0.00081 -- sklearn/preprocessing/_label.py:278:fit (fit)
isspmatrix -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix) +++
unique_labels -- 1 1 -- 0.00008 0.00048 -- sklearn/utils/multiclass.py:40:unique_labels (unique_labels)
<genexpr> -- 2 2 -- 0.00000 0.00025 -- sklearn/utils/multiclass.py:75:<genexpr> (<genexpr>)
type_of_target -- 1 1 -- 0.00004 0.00025 -- sklearn/utils/multiclass.py:200:type_of_target (type_of_target) +++
<genexpr> -- 2 2 -- 0.00000 0.00012 -- sklearn/utils/multiclass.py:103:<genexpr> (<genexpr>)
_unique_multiclass -- 1 1 -- 0.00001 0.00011 -- sklearn/utils/multiclass.py:22:_unique_multiclass (_unique_multiclass)
unique -- 1 1 -- 0.00000 0.00010 -- <__array_function__ internals>:177:unique (unique) +++
<genexpr> -- 3 3 -- 0.00000 0.00001 -- sklearn/utils/multiclass.py:106:<genexpr> (<genexpr>)
type_of_target -- 1 1 -- 0.00005 0.00028 -- sklearn/utils/multiclass.py:200:type_of_target (type_of_target) +++
_num_samples -- 1 1 -- 0.00002 0.00003 -- sklearn/utils/validation.py:254:_num_samples (_num_samples) +++
transform -- 1 1 -- 0.00002 0.00235 -- sklearn/preprocessing/_label.py:326:transform (transform)
label_binarize -- 1 1 -- 0.00014 0.00202 -- sklearn/preprocessing/_label.py:415:label_binarize (label_binarize)
in1d -- 1 1 -- 0.00000 0.00015 -- <__array_function__ internals>:177:in1d (in1d)
_in1d_dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/lib/arraysetops.py:515:_in1d_dispatcher (_in1d_dispatcher)
<built-in met...ay_function> -- 1 1 -- 0.00001 0.00014 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
sort -- 1 1 -- 0.00001 0.00004 -- <__array_function__ internals>:177:sort (sort)
_sort_dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:848:_sort_dispatcher (_sort_dispatcher)
searchsorted -- 1 1 -- 0.00001 0.00006 -- <__array_function__ internals>:177:searchsorted (searchsorted)
_searchsorted_dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:1315:_searchsorted_dispatcher (_searchsorted_dispatcher)
any -- 1 1 -- 0.00001 0.00007 -- <__array_function__ internals>:177:any (any) +++
cumsum -- 1 1 -- 0.00000 0.00007 -- <__array_function__ internals>:177:cumsum (cumsum)
_cumsum_dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:2491:_cumsum_dispatcher (_cumsum_dispatcher)
hstack -- 1 1 -- 0.00000 0.00011 -- <__array_function__ internals>:177:hstack (hstack)
_vhstack_dispatcher -- 1 1 -- 0.00000 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/shape_base.py:218:_vhstack_dispatcher (_vhstack_dispatcher)
_arrays_for...dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/shape_base.py:207:_arrays_for_stack_dispatcher (_arrays_for_stack_dispatcher)
empty_like -- 1 1 -- 0.00001 0.00001 -- <__array_function__ internals>:177:empty_like (empty_like) +++
isspmatrix -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix) +++
__init__ -- 1 1 -- 0.00004 0.00080 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_compressed.py:26:__init__ (__init__)
isspmatrix -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix) +++
check_format -- 1 1 -- 0.00015 0.00034 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_compressed.py:136:check_format (check_format)
get_shape -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:119:get_shape (get_shape) +++
prune -- 1 1 -- 0.00003 0.00007 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_compressed.py:1168:prune (prune)
_prune_array -- 2 2 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/_lib/_util.py:142:_prune_array (_prune_array)
get_shape -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:119:get_shape (get_shape) +++
nnz -- 4 4 -- 0.00001 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:291:nnz (nnz)
getnnz -- 4 4 -- 0.00002 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_compressed.py:108:getnnz (getnnz)
_swap -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_csr.py:231:_swap (_swap) +++
_swap -- 2 2 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_csr.py:231:_swap (_swap) +++
to_native -- 1 1 -- 0.00001 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_sputils.py:91:to_native (to_native)
get_index_dtype -- 1 1 -- 0.00003 0.00009 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_sputils.py:131:get_index_dtype (get_index_dtype) +++
__init__ -- 1 1 -- 0.00000 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_data.py:20:__init__ (__init__)
__init__ -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:105:__init__ (__init__)
get_index_dtype -- 1 1 -- 0.00008 0.00032 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_sputils.py:131:get_index_dtype (get_index_dtype) +++
isshape -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_sputils.py:220:isshape (isshape)
check_shape -- 1 1 -- 0.00003 0.00005 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_sputils.py:277:check_shape (check_shape)
<genexpr> -- 3 3 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_sputils.py:290:<genexpr> (<genexpr>)
toarray -- 1 1 -- 0.00003 0.00007 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_compressed.py:1048:toarray (toarray)
get_shape -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:119:get_shape (get_shape) +++
_process_toarray_args -- 1 1 -- 0.00001 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1277:_process_toarray_args (_process_toarray_args)
get_shape -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:119:get_shape (get_shape) +++
_get_dtype -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_data.py:23:_get_dtype (_get_dtype)
tocsr -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_csr.py:164:tocsr (tocsr)
_swap -- 2 2 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_csr.py:231:_swap (_swap) +++
type_of_target -- 1 1 -- 0.00004 0.00025 -- sklearn/utils/multiclass.py:200:type_of_target (type_of_target) +++
check_array -- 1 1 -- 0.00004 0.00017 -- sklearn/utils/validation.py:494:check_array (check_array) +++
column_or_1d -- 1 1 -- 0.00001 0.00005 -- sklearn/utils/validation.py:1002:column_or_1d (column_or_1d)
ravel -- 1 1 -- 0.00000 0.00002 -- <__array_function__ internals>:177:ravel (ravel)
_ravel_dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:1751:_ravel_dispatcher (_ravel_dispatcher)
shape -- 1 1 -- 0.00000 0.00001 -- <__array_function__ internals>:177:shape (shape)
_shape_dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:1961:_shape_dispatcher (_shape_dispatcher)
type_of_target -- 1 1 -- 0.00004 0.00026 -- sklearn/utils/multiclass.py:200:type_of_target (type_of_target) +++
check_is_fitted -- 1 1 -- 0.00002 0.00006 -- sklearn/utils/validation.py:1153:check_is_fitted (check_is_fitted)
isclass -- 1 1 -- 0.00000 0.00000 -- /usr/local/lib/python3.9/inspect.py:73:isclass (isclass)
<listcomp> -- 1 1 -- 0.00002 0.00003 -- sklearn/utils/validation.py:1217:<listcomp> (<listcomp>)
check_random_state -- 1 1 -- 0.00001 0.00001 -- sklearn/utils/validation.py:1043:check_random_state (check_random_state) +++
<built-in method builtins.print> -- 3 3 -- 0.00004 0.00228 -- ~:0:<built-in method builtins.print> (<built-in method builtins.print>)
write -- 6 6 -- 0.00010 0.00224 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx_gallery/gen_rst.py:81:write (write) +++
_bio_cache -- 320000 320000 -- 1.01550 1.25964 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:158:_bio_cache (_bio_cache)
<built-in method builtins.id> -- 320000 320000 -- 0.24414 0.24414 -- ~:0:<built-in method builtins.id> (<built-in method builtins.id>) +++
_bio_ptr -- 320000 320000 -- 1.63802 1.63802 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:175:_bio_ptr (_bio_ptr)
_bind_input_ortvalue -- 225000 225000 -- 1.10708 3.49690 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:179:_bind_input_ortvalue (_bind_input_ortvalue)
_bio_cache -- 225000 225000 -- 0.72184 0.89661 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:158:_bio_cache (_bio_cache) +++
_bio_do_bind_in -- 15614 15614 -- 0.20549 0.20549 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:171:_bio_do_bind_in (_bio_do_bind_in)
_bio_ptr -- 225000 225000 -- 1.16621 1.16621 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:175:_bio_ptr (_bio_ptr) +++
<built-in method builtins.isinstance> -- 225000 225000 -- 0.12150 0.12150 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
_bind_output_ortvalue -- 95000 95000 -- 0.49910 1.43974 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:225:_bind_output_ortvalue (_bind_output_ortvalue)
_bio_cache -- 95000 95000 -- 0.29367 0.36303 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:158:_bio_cache (_bio_cache) +++
_bio_ptr -- 95000 95000 -- 0.47181 0.47181 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:175:_bio_ptr (_bio_ptr) +++
_bio_do_bind_out -- 5017 5017 -- 0.05687 0.05687 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/_base_onnx_function.py:221:_bio_do_bind_out (_bio_do_bind_out)
<built-in method builtins.isinstance> -- 95000 95000 -- 0.04892 0.04892 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
_get_att_state -- 205 205 -- 0.00024 0.00024 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:139:_get_att_state (_get_att_state)
get_full_state -- 101 301 -- 0.00156 0.00327 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:147:get_full_state (get_full_state)
_get_att_state -- 201 201 -- 0.00023 0.00023 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:139:_get_att_state (_get_att_state) +++
<listcomp> -- 100 100 -- 0.00082 0.00247 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:152:<listcomp> (<listcomp>)
get_full_state -- 200 200 -- 0.00082 0.00165 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/optimizers_partial.py:147:get_full_state (get_full_state) +++
<built-in method builtins.getattr> -- 201 201 -- 0.00012 0.00012 -- ~:0:<built-in method builtins.getattr> (<built-in method builtins.getattr>) +++
<built-in method builtins.hasattr> -- 201 201 -- 0.00015 0.00015 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<built-in method builtins.isinstance> -- 301 301 -- 0.00039 0.00039 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
_provider_name_to_device_type -- 9 9 -- 0.00002 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:271:_provider_name_to_device_type (_provider_name_to_device_type)
get_initializer -- 14 14 -- 0.00039 0.00634 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:280:get_initializer (get_initializer)
to_array -- 12 12 -- 0.00100 0.00594 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/onnx/numpy_helper.py:21:to_array (to_array)
uses_external_data -- 12 12 -- 0.00004 0.00008 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/onnx/external_data_helper.py:224:uses_external_data (uses_external_data)
<method 'HasField' of '...age.CMessage' objects> -- 12 12 -- 0.00004 0.00004 -- ~:0:<method 'HasField' of 'google.protobuf.pyext._message.CMessage' objects> (<method 'HasField' of 'google.protobuf.pyext._message.CMessage' objects>) +++
<method 'HasField' of 'go...ssage.CMessage' objects> -- 24 24 -- 0.00008 0.00008 -- ~:0:<method 'HasField' of 'google.protobuf.pyext._message.CMessage' objects> (<method 'HasField' of 'google.protobuf.pyext._message.CMessage' objects>) +++
<method 'astype' of 'numpy.ndarray' objects> -- 12 12 -- 0.00011 0.00011 -- ~:0:<method 'astype' of 'numpy.ndarray' objects> (<method 'astype' of 'numpy.ndarray' objects>) +++
<method 'reshape' of 'numpy.ndarray' objects> -- 12 12 -- 0.00009 0.00009 -- ~:0:<method 'reshape' of 'numpy.ndarray' objects> (<method 'reshape' of 'numpy.ndarray' objects>) +++
<built-in method numpy.asarray> -- 12 12 -- 0.00455 0.00455 -- ~:0:<built-in method numpy.asarray> (<built-in method numpy.asarray>) +++
<built-in method builtins.getattr> -- 12 12 -- 0.00003 0.00003 -- ~:0:<built-in method builtins.getattr> (<built-in method builtins.getattr>) +++
input_to_ort -- 10000 10000 -- 0.35384 0.48738 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:578:input_to_ort (input_to_ort)
<built-in method builtins.all> -- 10000 10000 -- 0.04446 0.09059 -- ~:0:<built-in method builtins.all> (<built-in method builtins.all>) +++
<built-in method builtins.isinstance> -- 10000 10000 -- 0.03533 0.03533 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.len> -- 10000 10000 -- 0.00762 0.00762 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
_call_iobinding -- 35000 35000 -- 2.54616 2.54616 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_penalty.py:27:_call_iobinding (_call_iobinding)
value -- 30100 30100 -- 0.03640 0.03640 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/sgd_learning_rate.py:186:value (value)
_mean -- 15100 15100 -- 0.72528 1.45931 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:162:_mean (_mean)
_count_reduce_items -- 15100 15100 -- 0.27440 0.31784 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:66:_count_reduce_items (_count_reduce_items)
<built-in method numpy.co...th.normalize_axis_index> -- 15200 15200 -- 0.02385 0.02385 -- ~:0:<built-in method numpy.core._multiarray_umath.normalize_axis_index> (<built-in method numpy.core._multiarray_umath.normalize_axis_index>)
<built-in method builtins.isinstance> -- 15000 15000 -- 0.01959 0.01959 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method numpy.asanyarray> -- 15100 15100 -- 0.01208 0.01208 -- ~:0:<built-in method numpy.asanyarray> (<built-in method numpy.asanyarray>) +++
<method 'reduce' of 'numpy.ufunc' objects> -- 15100 15100 -- 0.36575 0.36575 -- ~:0:<method 'reduce' of 'numpy.ufunc' objects> (<method 'reduce' of 'numpy.ufunc' objects>) +++
<built-in method builtins.hasattr> -- 100 100 -- 0.00020 0.00020 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<built-in method builtins.isinstance> -- 15100 15100 -- 0.01086 0.01086 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.issubclass> -- 30200 30200 -- 0.02730 0.02730 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>) +++
_wrapfunc -- 5003 5003 -- 0.02595 0.84355 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:51:_wrapfunc (_wrapfunc)
_wrapit -- 1 1 -- 0.00002 0.00019 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:38:_wrapit (_wrapit)
<method 'clip' of 'numpy.ndarray' objects> -- 1 1 -- 0.00001 0.00016 -- ~:0:<method 'clip' of 'numpy.ndarray' objects> (<method 'clip' of 'numpy.ndarray' objects>) +++
<method 'clip' of 'numpy.ndarray' objects> -- 5000 5000 -- 0.02850 0.80698 -- ~:0:<method 'clip' of 'numpy.ndarray' objects> (<method 'clip' of 'numpy.ndarray' objects>) +++
<built-in method builtins.getattr> -- 5003 5003 -- 0.01035 0.01035 -- ~:0:<built-in method builtins.getattr> (<built-in method builtins.getattr>) +++
_wrapreduction -- 3 3 -- 0.00005 0.00038 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:69:_wrapreduction (_wrapreduction)
<dictcomp> -- 3 3 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:70:<dictcomp> (<dictcomp>)
<method 'reduce' of 'numpy.ufunc' objects> -- 3 3 -- 0.00032 0.00032 -- ~:0:<method 'reduce' of 'numpy.ufunc' objects> (<method 'reduce' of 'numpy.ufunc' objects>) +++
issubdtype -- 3 3 -- 0.00002 0.00006 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/numerictypes.py:356:issubdtype (issubdtype)
issubclass_ -- 6 6 -- 0.00002 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/numerictypes.py:282:issubclass_ (issubclass_)
get_shape -- 4 4 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:119:get_shape (get_shape)
isspmatrix -- 45210 45210 -- 0.07292 0.13031 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix)
<built-in method builtins.isinstance> -- 45210 45210 -- 0.05740 0.05740 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
_swap -- 5 5 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_csr.py:231:_swap (_swap)
get_index_dtype -- 2 2 -- 0.00011 0.00041 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_sputils.py:131:get_index_dtype (get_index_dtype)
can_cast -- 4 4 -- 0.00002 0.00006 -- <__array_function__ internals>:177:can_cast (can_cast)
can_cast -- 4 4 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/multiarray.py:498:can_cast (can_cast)
__init__ -- 4 4 -- 0.00005 0.00005 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/getlimits.py:647:__init__ (__init__)
min -- 2 2 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/getlimits.py:658:min (min)
max -- 2 2 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/getlimits.py:671:max (max)
issubdtype -- 2 2 -- 0.00001 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/numerictypes.py:356:issubdtype (issubdtype) +++
write -- 7 7 -- 0.00013 0.00324 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx_gallery/gen_rst.py:81:write (write)
verbose -- 6 6 -- 0.00005 0.00309 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:135:verbose (verbose)
log -- 6 6 -- 0.00008 0.00304 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:128:log (log)
log -- 6 6 -- 0.00009 0.00296 -- /usr/local/lib/python3.9/logging/__init__.py:1825:log (log)
log -- 6 6 -- 0.00008 0.00278 -- /usr/local/lib/python3.9/logging/__init__.py:1485:log (log)
_log -- 6 6 -- 0.00006 0.00269 -- /usr/local/lib/python3.9/logging/__init__.py:1553:_log (_log)
findCaller -- 6 6 -- 0.00009 0.00017 -- /usr/local/lib/python3.9/logging/__init__.py:1502:findCaller (findCaller)
<lambda> -- 6 6 -- 0.00002 0.00003 -- /usr/local/lib/python3.9/logging/__init__.py:156:<lambda> (<lambda>)
normcase -- 12 12 -- 0.00002 0.00003 -- /usr/local/lib/python3.9/posixpath.py:52:normcase (normcase)
<built-in met...osix.fspath> -- 12 12 -- 0.00001 0.00001 -- ~:0:<built-in method posix.fspath> (<built-in method posix.fspath>) +++
<built-in metho...ltins.hasattr> -- 12 12 -- 0.00001 0.00001 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
makeRecord -- 6 6 -- 0.00006 0.00078 -- /usr/local/lib/python3.9/logging/__init__.py:1538:makeRecord (makeRecord)
__init__ -- 6 6 -- 0.00031 0.00073 -- /usr/local/lib/python3.9/logging/__init__.py:278:__init__ (__init__)
getLevelName -- 6 6 -- 0.00005 0.00005 -- /usr/local/lib/python3.9/logging/__init__.py:119:getLevelName (getLevelName)
<method 'ge...' objects> -- 12 12 -- 0.00001 0.00001 -- ~:0:<method 'get' of 'dict' objects> (<method 'get' of 'dict' objects>) +++
current_process -- 6 6 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/multiprocessing/process.py:37:current_process (current_process)
name -- 6 6 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/multiprocessing/process.py:189:name (name)
splitext -- 6 6 -- 0.00003 0.00010 -- /usr/local/lib/python3.9/posixpath.py:117:splitext (splitext)
_splitext -- 6 6 -- 0.00004 0.00006 -- /usr/local/lib/python3.9/genericpath.py:121:_splitext (_splitext)
<method '...objects> -- 12 12 -- 0.00001 0.00001 -- ~:0:<method 'rfind' of 'str' objects> (<method 'rfind' of 'str' objects>) +++
basename -- 6 6 -- 0.00005 0.00009 -- /usr/local/lib/python3.9/posixpath.py:140:basename (basename)
_get_sep -- 6 6 -- 0.00001 0.00002 -- /usr/local/lib/python3.9/posixpath.py:41:_get_sep (_get_sep)
name -- 6 6 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/threading.py:1053:name (name)
current_thread -- 6 6 -- 0.00002 0.00002 -- /usr/local/lib/python3.9/threading.py:1318:current_thread (current_thread)
handle -- 6 6 -- 0.00002 0.00168 -- /usr/local/lib/python3.9/logging/__init__.py:1579:handle (handle)
filter -- 6 6 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/logging/__init__.py:787:filter (filter) +++
callHandlers -- 6 6 -- 0.00007 0.00164 -- /usr/local/lib/python3.9/logging/__init__.py:1633:callHandlers (callHandlers)
handle -- 12 12 -- 0.00008 0.00157 -- /usr/local/lib/python3.9/logging/__init__.py:935:handle (handle)
filter -- 12 12 -- 0.00006 0.00017 -- /usr/local/lib/python3.9/logging/__init__.py:787:filter (filter) +++
acquire -- 12 12 -- 0.00003 0.00006 -- /usr/local/lib/python3.9/logging/__init__.py:892:acquire (acquire) +++
release -- 12 12 -- 0.00002 0.00003 -- /usr/local/lib/python3.9/logging/__init__.py:899:release (release) +++
emit -- 6 6 -- 0.00004 0.00040 -- /usr/local/lib/python3.9/logging/__init__.py:1067:emit (emit) +++
emit -- 6 6 -- 0.00006 0.00083 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:158:emit (emit)
acquire -- 6 6 -- 0.00001 0.00002 -- /usr/local/lib/python3.9/logging/__init__.py:892:acquire (acquire) +++
release -- 6 6 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/logging/__init__.py:899:release (release) +++
emit -- 6 6 -- 0.00005 0.00073 -- /usr/local/lib/python3.9/logging/__init__.py:1067:emit (emit) +++
isEnabledFor -- 6 6 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/logging/__init__.py:1677:isEnabledFor (isEnabledFor) +++
isEnabledFor -- 6 6 -- 0.00002 0.00003 -- /usr/local/lib/python3.9/logging/__init__.py:1834:isEnabledFor (isEnabledFor)
isEnabledFor -- 6 6 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/logging/__init__.py:1677:isEnabledFor (isEnabledFor) +++
process -- 6 6 -- 0.00005 0.00006 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sphinx/util/logging.py:138:process (process)
numpy_to_ort_value -- 10026 10026 -- 0.03218 0.15713 -- onnxruntime_helper.py:134:numpy_to_ort_value (numpy_to_ort_value)
<built-in method onnxruntim...state.ortvalue_from_numpy> -- 10026 10026 -- 0.12495 0.12495 -- ~:0:<built-in method onnxruntime.capi.onnxruntime_pybind11_state.ortvalue_from_numpy> (<built-in method onnxruntime.capi.onnxruntime_pybind11_state.ortvalue_from_numpy>) +++
_safe_indexing -- 5100 5100 -- 0.05663 1.03506 -- sklearn/utils/__init__.py:307:_safe_indexing (_safe_indexing)
_array_indexing -- 5100 5100 -- 0.20046 0.83872 -- sklearn/utils/__init__.py:193:_array_indexing (_array_indexing)
isspmatrix -- 5100 5100 -- 0.00730 0.01271 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix) +++
parse -- 5100 5100 -- 0.02751 0.60001 -- sklearn/externals/_packaging/version.py:65:parse (parse)
__init__ -- 5100 5100 -- 0.23946 0.57251 -- sklearn/externals/_packaging/version.py:284:__init__ (__init__)
<lambda> -- 5100 5100 -- 0.01281 0.02426 -- <string>:1:<lambda> (<lambda>)
<built-in method __...at 0x7f5da6713960> -- 5100 5100 -- 0.01145 0.01145 -- ~:0:<built-in method __new__ of type object at 0x7f5da6713960> (<built-in method __new__ of type object at 0x7f5da6713960>)
<genexpr> -- 15300 15300 -- 0.04081 0.04081 -- sklearn/externals/_packaging/version.py:294:<genexpr> (<genexpr>)
_parse_letter_version -- 15300 15300 -- 0.01230 0.01230 -- sklearn/externals/_packaging/version.py:416:_parse_letter_version (_parse_letter_version)
_parse_local_version -- 5100 5100 -- 0.00353 0.00353 -- sklearn/externals/_packaging/version.py:455:_parse_local_version (_parse_local_version)
_cmpkey -- 5100 5100 -- 0.08785 0.09319 -- sklearn/externals/_packaging/version.py:467:_cmpkey (_cmpkey)
<lambda> -- 5100 5100 -- 0.00533 0.00533 -- sklearn/externals/_packaging/version.py:482:<lambda> (<lambda>)
<method 'split' of 'str' objects> -- 5100 5100 -- 0.01298 0.01298 -- ~:0:<method 'split' of 'str' objects> (<method 'split' of 'str' objects>)
<method 'group' of 're.Match' objects> -- 51000 51000 -- 0.05913 0.05913 -- ~:0:<method 'group' of 're.Match' objects> (<method 'group' of 're.Match' objects>)
<method 'search' of 're.Pattern' objects> -- 5100 5100 -- 0.08686 0.08686 -- ~:0:<method 'search' of 're.Pattern' objects> (<method 'search' of 're.Pattern' objects>)
__lt__ -- 5100 5100 -- 0.01831 0.02272 -- sklearn/externals/_packaging/version.py:92:__lt__ (__lt__)
<built-in method builtins.isinstance> -- 5100 5100 -- 0.00440 0.00440 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.isinstance> -- 5100 5100 -- 0.00282 0.00282 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
_determine_key_type -- 5100 5100 -- 0.09773 0.12558 -- sklearn/utils/__init__.py:237:_determine_key_type (_determine_key_type)
<method 'keys' of 'dict' objects> -- 5100 5100 -- 0.00580 0.00580 -- ~:0:<method 'keys' of 'dict' objects> (<method 'keys' of 'dict' objects>)
<built-in method builtins.hasattr> -- 5100 5100 -- 0.00446 0.00446 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<built-in method builtins.isinstance> -- 15300 15300 -- 0.01758 0.01758 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.hasattr> -- 10200 10200 -- 0.01413 0.01413 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
safe_sparse_dot -- 40000 40000 -- 1.62780 1.74506 -- sklearn/utils/extmath.py:120:safe_sparse_dot (safe_sparse_dot)
isspmatrix -- 40000 40000 -- 0.06544 0.11727 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix) +++
type_of_target -- 4 4 -- 0.00016 0.00104 -- sklearn/utils/multiclass.py:200:type_of_target (type_of_target)
simplefilter -- 4 4 -- 0.00002 0.00008 -- /usr/local/lib/python3.9/warnings.py:165:simplefilter (simplefilter) +++
__init__ -- 4 4 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/warnings.py:437:__init__ (__init__) +++
__enter__ -- 4 4 -- 0.00002 0.00003 -- /usr/local/lib/python3.9/warnings.py:458:__enter__ (__enter__) +++
__exit__ -- 4 4 -- 0.00002 0.00002 -- /usr/local/lib/python3.9/warnings.py:477:__exit__ (__exit__) +++
unique -- 4 4 -- 0.00002 0.00044 -- <__array_function__ internals>:177:unique (unique) +++
isspmatrix -- 4 4 -- 0.00000 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix) +++
is_multilabel -- 4 4 -- 0.00008 0.00024 -- sklearn/utils/multiclass.py:116:is_multilabel (is_multilabel)
simplefilter -- 4 4 -- 0.00002 0.00009 -- /usr/local/lib/python3.9/warnings.py:165:simplefilter (simplefilter) +++
__init__ -- 4 4 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/warnings.py:437:__init__ (__init__) +++
__enter__ -- 4 4 -- 0.00003 0.00003 -- /usr/local/lib/python3.9/warnings.py:458:__enter__ (__enter__) +++
__exit__ -- 4 4 -- 0.00002 0.00002 -- /usr/local/lib/python3.9/warnings.py:477:__exit__ (__exit__) +++
_num_samples -- 106 106 -- 0.00242 0.00459 -- sklearn/utils/validation.py:254:_num_samples (_num_samples)
<built-in method builtins.hasattr> -- 318 318 -- 0.00048 0.00048 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>) +++
<built-in method builtins.isinstance> -- 106 106 -- 0.00031 0.00163 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.len> -- 106 106 -- 0.00007 0.00007 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
check_consistent_length -- 101 101 -- 0.00122 0.01742 -- sklearn/utils/validation.py:318:check_consistent_length (check_consistent_length)
unique -- 101 101 -- 0.00062 0.01130 -- <__array_function__ internals>:177:unique (unique) +++
<listcomp> -- 101 101 -- 0.00040 0.00483 -- sklearn/utils/validation.py:329:<listcomp> (<listcomp>)
_num_samples -- 102 102 -- 0.00235 0.00443 -- sklearn/utils/validation.py:254:_num_samples (_num_samples) +++
<built-in method builtins.len> -- 101 101 -- 0.00007 0.00007 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
check_array -- 3 3 -- 0.00014 0.00102 -- sklearn/utils/validation.py:494:check_array (check_array)
simplefilter -- 3 3 -- 0.00002 0.00009 -- /usr/local/lib/python3.9/warnings.py:165:simplefilter (simplefilter) +++
__init__ -- 3 3 -- 0.00001 0.00001 -- /usr/local/lib/python3.9/warnings.py:437:__init__ (__init__) +++
__enter__ -- 3 3 -- 0.00004 0.00004 -- /usr/local/lib/python3.9/warnings.py:458:__enter__ (__enter__) +++
__exit__ -- 3 3 -- 0.00002 0.00002 -- /usr/local/lib/python3.9/warnings.py:477:__exit__ (__exit__) +++
isspmatrix -- 3 3 -- 0.00000 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/scipy/sparse/_base.py:1291:isspmatrix (isspmatrix) +++
_assert_all_finite -- 3 3 -- 0.00014 0.00055 -- sklearn/utils/validation.py:90:_assert_all_finite (_assert_all_finite)
get_config -- 3 3 -- 0.00002 0.00003 -- sklearn/_config.py:24:get_config (get_config)
_get_threadlocal_config -- 3 3 -- 0.00001 0.00001 -- sklearn/_config.py:16:_get_threadlocal_config (_get_threadlocal_config)
_safe_accumulator_op -- 1 1 -- 0.00002 0.00035 -- sklearn/utils/extmath.py:869:_safe_accumulator_op (_safe_accumulator_op)
sum -- 1 1 -- 0.00001 0.00030 -- <__array_function__ internals>:177:sum (sum)
_sum_dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:2155:_sum_dispatcher (_sum_dispatcher)
<built-in method nump...ment_array_function> -- 1 1 -- 0.00001 0.00030 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
issubdtype -- 1 1 -- 0.00001 0.00002 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/numerictypes.py:356:issubdtype (issubdtype) +++
_num_samples -- 3 3 -- 0.00006 0.00012 -- sklearn/utils/validation.py:254:_num_samples (_num_samples) +++
_ensure_no_complex_data -- 3 3 -- 0.00001 0.00001 -- sklearn/utils/validation.py:484:_ensure_no_complex_data (_ensure_no_complex_data)
check_random_state -- 101 101 -- 0.00096 0.00247 -- sklearn/utils/validation.py:1043:check_random_state (check_random_state)
<built-in method builtins.isinstance> -- 200 200 -- 0.00063 0.00150 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method builtins.len> -- 60991 60991 -- 0.08205 0.10510 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>)
__len__ -- 10200 10200 -- 0.02305 0.02305 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/data_loader.py:94:__len__ (__len__)
<method 'astype' of 'numpy.ndarray' objects> -- 26 26 -- 0.00026 0.00026 -- ~:0:<method 'astype' of 'numpy.ndarray' objects> (<method 'astype' of 'numpy.ndarray' objects>)
<method 'append' of 'list' objects> -- 10283 10283 -- 0.01700 0.01700 -- ~:0:<method 'append' of 'list' objects> (<method 'append' of 'list' objects>)
<built-in method builtins.hasattr> -- 156138 156138 -- 0.13126 0.13126 -- ~:0:<built-in method builtins.hasattr> (<built-in method builtins.hasattr>)
<built-in method builtins.isinstance> -- 481660 481660 -- 0.34062 0.34361 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>)
__instancecheck__ -- 320 320 -- 0.00050 0.00298 -- /usr/local/lib/python3.9/abc.py:96:__instancecheck__ (__instancecheck__)
<built-in method _abc._abc_instancecheck> -- 320 320 -- 0.00182 0.00249 -- ~:0:<built-in method _abc._abc_instancecheck> (<built-in method _abc._abc_instancecheck>)
__subclasscheck__ -- 106 106 -- 0.00019 0.00066 -- /usr/local/lib/python3.9/abc.py:100:__subclasscheck__ (__subclasscheck__)
<built-in method _abc._abc_subclasscheck> -- 106 106 -- 0.00048 0.00048 -- ~:0:<built-in method _abc._abc_subclasscheck> (<built-in method _abc._abc_subclasscheck>)
<method 'reshape' of 'numpy.ndarray' objects> -- 16 16 -- 0.00012 0.00012 -- ~:0:<method 'reshape' of 'numpy.ndarray' objects> (<method 'reshape' of 'numpy.ndarray' objects>)
<built-in method builtins.getattr> -- 5257 5257 -- 0.01054 0.01054 -- ~:0:<built-in method builtins.getattr> (<built-in method builtins.getattr>)
<built-in method numpy.array> -- 105 105 -- 0.00859 0.00859 -- ~:0:<built-in method numpy.array> (<built-in method numpy.array>)
<built-in method numpy.zeros> -- 20 20 -- 0.00014 0.00014 -- ~:0:<built-in method numpy.zeros> (<built-in method numpy.zeros>)
<built-in method onnxruntime....1_state.ortvalue_from_numpy> -- 70026 70026 -- 0.85450 0.85450 -- ~:0:<built-in method onnxruntime.capi.onnxruntime_pybind11_state.ortvalue_from_numpy> (<built-in method onnxruntime.capi.onnxruntime_pybind11_state.ortvalue_from_numpy>)
<built-in method builtins.id> -- 325001 325001 -- 0.25124 0.25124 -- ~:0:<built-in method builtins.id> (<built-in method builtins.id>)
<built-in method numpy.empty> -- 112 112 -- 0.00065 0.00065 -- ~:0:<built-in method numpy.empty> (<built-in method numpy.empty>)
<built-in method numpy.arange> -- 101 101 -- 0.00149 0.00149 -- ~:0:<built-in method numpy.arange> (<built-in method numpy.arange>)
<built-in method numpy.core._...th.implement_array_function> -- 35128 45144 -- 0.43922 2.84274 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>)
sort -- 1 1 -- 0.00001 0.00003 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:852:sort (sort)
searchsorted -- 1 1 -- 0.00001 0.00005 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:1319:searchsorted (searchsorted)
_wrapfunc -- 1 1 -- 0.00001 0.00004 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:51:_wrapfunc (_wrapfunc) +++
ravel -- 1 1 -- 0.00001 0.00001 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:1755:ravel (ravel)
shape -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:1965:shape (shape)
clip -- 5001 5001 -- 0.03834 0.88179 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:2083:clip (clip)
_wrapfunc -- 5001 5001 -- 0.02593 0.84344 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:51:_wrapfunc (_wrapfunc) +++
sum -- 1 1 -- 0.00001 0.00029 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:2160:sum (sum)
_wrapreduction -- 1 1 -- 0.00001 0.00028 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:69:_wrapreduction (_wrapreduction) +++
any -- 2 2 -- 0.00002 0.00013 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:2305:any (any)
_wrapreduction -- 2 2 -- 0.00004 0.00011 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:69:_wrapreduction (_wrapreduction) +++
cumsum -- 1 1 -- 0.00001 0.00006 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:2495:cumsum (cumsum)
_wrapfunc -- 1 1 -- 0.00001 0.00006 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:51:_wrapfunc (_wrapfunc) +++
ndim -- 10002 10002 -- 0.01796 0.01797 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:3164:ndim (ndim)
mean -- 15000 15000 -- 0.15178 1.59581 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:3356:mean (mean)
_mean -- 15000 15000 -- 0.71810 1.44402 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:162:_mean (_mean) +++
zeros_like -- 6 6 -- 0.00007 0.00032 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/numeric.py:76:zeros_like (zeros_like)
empty_like -- 6 6 -- 0.00004 0.00010 -- <__array_function__ internals>:177:empty_like (empty_like) +++
copyto -- 6 6 -- 0.00004 0.00010 -- <__array_function__ internals>:177:copyto (copyto)
copyto -- 6 6 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/multiarray.py:1071:copyto (copyto)
atleast_1d -- 1 1 -- 0.00002 0.00004 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/shape_base.py:23:atleast_1d (atleast_1d)
hstack -- 1 1 -- 0.00001 0.00009 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/shape_base.py:285:hstack (hstack)
atleast_1d -- 1 1 -- 0.00000 0.00005 -- <__array_function__ internals>:177:atleast_1d (atleast_1d)
_atleast_1d_dispatcher -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/shape_base.py:19:_atleast_1d_dispatcher (_atleast_1d_dispatcher)
concatenate -- 1 1 -- 0.00001 0.00003 -- <__array_function__ internals>:177:concatenate (concatenate)
concatenate -- 1 1 -- 0.00000 0.00000 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/multiarray.py:148:concatenate (concatenate)
unique -- 106 106 -- 0.00101 0.01031 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/lib/arraysetops.py:138:unique (unique)
_unpack_tuple -- 106 106 -- 0.00026 0.00033 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/lib/arraysetops.py:125:_unpack_tuple (_unpack_tuple)
<built-in method builtins.len> -- 106 106 -- 0.00007 0.00007 -- ~:0:<built-in method builtins.len> (<built-in method builtins.len>) +++
_unique1d -- 106 106 -- 0.00613 0.00794 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/lib/arraysetops.py:320:_unique1d (_unique1d)
<method 'flatten' of 'numpy.ndarray' objects> -- 106 106 -- 0.00084 0.00084 -- ~:0:<method 'flatten' of 'numpy.ndarray' objects> (<method 'flatten' of 'numpy.ndarray' objects>)
<method 'sort' of 'numpy.ndarray' objects> -- 106 106 -- 0.00031 0.00031 -- ~:0:<method 'sort' of 'numpy.ndarray' objects> (<method 'sort' of 'numpy.ndarray' objects>) +++
<built-in method numpy.asanyarray> -- 106 106 -- 0.00005 0.00005 -- ~:0:<built-in method numpy.asanyarray> (<built-in method numpy.asanyarray>) +++
<built-in method numpy.empty> -- 106 106 -- 0.00062 0.00062 -- ~:0:<built-in method numpy.empty> (<built-in method numpy.empty>) +++
<built-in method numpy.asanyarray> -- 106 106 -- 0.00103 0.00103 -- ~:0:<built-in method numpy.asanyarray> (<built-in method numpy.asanyarray>) +++
in1d -- 1 1 -- 0.00012 0.00014 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/lib/arraysetops.py:519:in1d (in1d)
<method 'HasField' of 'google...._message.CMessage' objects> -- 36 36 -- 0.00012 0.00012 -- ~:0:<method 'HasField' of 'google.protobuf.pyext._message.CMessage' objects> (<method 'HasField' of 'google.protobuf.pyext._message.CMessage' objects>)
<built-in method numpy.asarray> -- 38 38 -- 0.00458 0.00458 -- ~:0:<built-in method numpy.asarray> (<built-in method numpy.asarray>)
<built-in method builtins.all> -- 10004 10004 -- 0.04447 0.09059 -- ~:0:<built-in method builtins.all> (<built-in method builtins.all>)
<lambda> -- 40000 40000 -- 0.03384 0.04612 -- onnxcustom/onnxcustom_UT_39_std/_doc/sphinxdoc/source/onnxcustom/training/ortgradient.py:598:<lambda> (<lambda>)
<built-in method builtins.isinstance> -- 40000 40000 -- 0.01229 0.01229 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
<built-in method numpy.asanyarray> -- 15319 15319 -- 0.01317 0.01317 -- ~:0:<built-in method numpy.asanyarray> (<built-in method numpy.asanyarray>)
<method 'reduce' of 'numpy.ufunc' objects> -- 25107 25107 -- 0.60839 0.60839 -- ~:0:<method 'reduce' of 'numpy.ufunc' objects> (<method 'reduce' of 'numpy.ufunc' objects>)
<built-in method builtins.issubclass> -- 30209 30209 -- 0.02731 0.02731 -- ~:0:<built-in method builtins.issubclass> (<built-in method builtins.issubclass>)
<method 'ravel' of 'numpy.ndarray' objects> -- 15003 15003 -- 0.03573 0.03573 -- ~:0:<method 'ravel' of 'numpy.ndarray' objects> (<method 'ravel' of 'numpy.ndarray' objects>)
<method 'get' of 'dict' objects> -- 5034 5034 -- 0.00931 0.00931 -- ~:0:<method 'get' of 'dict' objects> (<method 'get' of 'dict' objects>)
<method 'sort' of 'numpy.ndarray' objects> -- 107 107 -- 0.00032 0.00032 -- ~:0:<method 'sort' of 'numpy.ndarray' objects> (<method 'sort' of 'numpy.ndarray' objects>)
<built-in method _warnings._filters_mutated> -- 33 33 -- 0.00002 0.00002 -- ~:0:<built-in method _warnings._filters_mutated> (<built-in method _warnings._filters_mutated>)
<method 'clip' of 'numpy.ndarray' objects> -- 5001 5001 -- 0.02851 0.80713 -- ~:0:<method 'clip' of 'numpy.ndarray' objects> (<method 'clip' of 'numpy.ndarray' objects>)
_clip -- 5001 5001 -- 0.08146 0.77863 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:125:_clip (_clip)
_clip_dep_is_scalar_nan -- 10002 10002 -- 0.33568 0.48824 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:91:_clip_dep_is_scalar_nan (_clip_dep_is_scalar_nan)
ndim -- 10002 10002 -- 0.04046 0.15256 -- <__array_function__ internals>:177:ndim (ndim)
_ndim_dispatcher -- 10002 10002 -- 0.00877 0.00877 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/fromnumeric.py:3160:_ndim_dispatcher (_ndim_dispatcher)
<built-in method nump...ment_array_function> -- 10002 10002 -- 0.08536 0.10333 -- ~:0:<built-in method numpy.core._multiarray_umath.implement_array_function> (<built-in method numpy.core._multiarray_umath.implement_array_function>) +++
_clip_dep_is_byte_swapped -- 10002 10002 -- 0.02773 0.03583 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:101:_clip_dep_is_byte_swapped (_clip_dep_is_byte_swapped)
<built-in method builtins.isinstance> -- 10002 10002 -- 0.00810 0.00810 -- ~:0:<built-in method builtins.isinstance> (<built-in method builtins.isinstance>) +++
_clip_dep_invoke_with_casting -- 5001 5001 -- 0.17309 0.17309 -- onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/numpy/core/_methods.py:106:_clip_dep_invoke_with_casting (_clip_dep_invoke_with_casting)
<built-in method posix.fspath> -- 24 24 -- 0.00001 0.00001 -- ~:0:<built-in method posix.fspath> (<built-in method posix.fspath>)
<built-in method _thread.get_ident> -- 12 12 -- 0.00001 0.00001 -- ~:0:<built-in method _thread.get_ident> (<built-in method _thread.get_ident>)
<method 'rfind' of 'str' objects> -- 18 18 -- 0.00003 0.00003 -- ~:0:<method 'rfind' of 'str' objects> (<method 'rfind' of 'str' objects>)
if GPU is available¶
if get_device().upper() == 'GPU':
train_session = OrtGradientForwardBackwardOptimizer(
onx, device='cuda', warm_start=False,
max_iter=max_iter, batch_size=batch_size,
learning_loss=NegLogLearningLoss(),
learning_rate=LearningRateSGDNesterov(
1e-7, nesterov=False, momentum=0.9),
learning_penalty=ElasticLearningPenalty(l1=0, l2=1e-4))
benches.append(benchmark(X_train, y_train, nn,
train_session, name='NN-GPU'))
A simple linear layer¶
nn = MLPClassifier(hidden_layer_sizes=tuple(), max_iter=max_iter,
solver='sgd', learning_rate_init=1e-1, alpha=1e-4,
n_iter_no_change=max_iter * 3, batch_size=batch_size,
nesterovs_momentum=True, momentum=0.9,
learning_rate="invscaling", activation='identity')
with warnings.catch_warnings():
warnings.simplefilter('ignore')
nn.fit(X_train, y_train)
onx = to_onnx(nn, X_train[:1].astype(numpy.float32), target_opset=15,
options={'zipmap': False, 'nocl': True})
onx = select_model_inputs_outputs(
onx, outputs=["add_result"], infer_shapes=True)
onx = onnx_rename_weights(onx)
try:
print(onnx_simple_text_plot(onx))
except RuntimeError as e:
print("You should upgrade mlprodict.")
print(e)
train_session = OrtGradientForwardBackwardOptimizer(
onx, device='cpu', warm_start=False,
max_iter=max_iter, batch_size=batch_size,
learning_loss=NegLogLearningLoss(),
learning_rate=LearningRateSGDNesterov(
1e-5, nesterov=True, momentum=0.9),
learning_penalty=ElasticLearningPenalty(l1=0, l2=1e-4))
benches.append(benchmark(X_train, y_train, nn, train_session, name='LR-CPU'))
if get_device().upper() == 'GPU':
train_session = OrtGradientForwardBackwardOptimizer(
onx, device='cuda', warm_start=False,
max_iter=max_iter, batch_size=batch_size,
learning_loss=NegLogLearningLoss(),
learning_rate=LearningRateSGDNesterov(
1e-5, nesterov=False, momentum=0.9),
learning_penalty=ElasticLearningPenalty(l1=0, l2=1e-4))
benches.append(benchmark(X_train, y_train, nn,
train_session, name='LR-GPU'))
Out:
opset: domain='' version=13
opset: domain='ai.onnx.ml' version=1
input: name='X' type=dtype('float32') shape=(0, 100)
init: name='I0_coefficient' type=dtype('float32') shape=(100,)
init: name='I1_intercepts' type=dtype('float32') shape=(1,) -- array([0.03688538], dtype=float32)
Cast(X, to=1) -> r1
MatMul(r1, I0_coefficient) -> r0
Add(r0, I1_intercepts) -> add_result
output: name='add_result' type=dtype('float32') shape=(0, 1)
[benchmark] LR-CPU
/var/lib/jenkins/workspace/onnxcustom/onnxcustom_UT_39_std/_venv/lib/python3.9/site-packages/sklearn/neural_network/_multilayer_perceptron.py:692: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (100) reached and the optimization hasn't converged yet.
warnings.warn(
[benchmark] skl=100 iterations - 5.613140130415559 seconds
[benchmark] ort=100 iteration - 7.722520655952394 seconds
Graphs¶
Dataframe first.
df = DataFrame(benches).set_index('name')
df
text output
print(df)
Out:
skl ... losses_ort
name ...
NN-CPU 12.829431 ... [6.6074824, 6.316644, 6.900415, 6.697754, 6.46...
LR-CPU 5.613140 ... [2.4641361, 2.9571128, 2.4631786, 2.4621465, 2...
[2 rows x 6 columns]
Graphs.
fig, ax = plt.subplots(1, 2, figsize=(10, 4))
df[['skl', 'ort']].plot.bar(title="Processing time", ax=ax[0])
ax[0].tick_params(axis='x', rotation=30)
for bench in benches:
ax[1].plot(bench['losses_skl'][1:], label='skl-' + bench['name'])
ax[1].plot(bench['losses_ort'][1:], label='ort-' + bench['name'])
ax[1].set_yscale('log')
ax[1].set_title("Losses")
ax[1].legend()
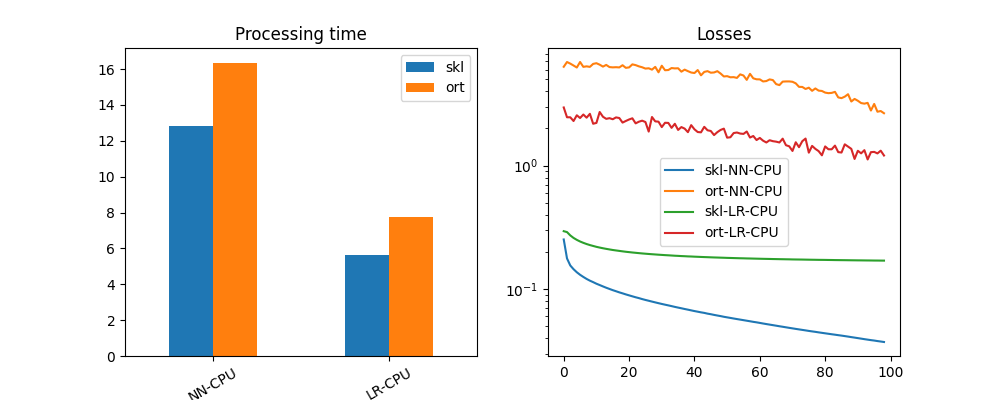
Out:
<matplotlib.legend.Legend object at 0x7f5cdc06d0a0>
The gradient update are not exactly the same. It should be improved for a fair comprison.
# plt.show()
Total running time of the script: ( 1 minutes 37.506 seconds)